Multilingual FAQ Bot Using RAG with Orq.ai
This cookbook covers how to leverage Orq.ai’s RAG and Routing Engine to build scalable, context-driven AI solutions.
This guide walks you through building a multilingual FAQ bot powered by Retrieval-Augmented Generation (RAG) and a Routing Engine on Orq.ai. The bot retrieves answers from a knowledge base in real-time and adapts responses to different languages based on user input.
Instead of managing multiple versions of your bot with hardcoded if-else statements, Orq.ai’s Routing Engine offers a centralized way to handle various chatbot variants. With the Routing Engine, you can:
✅ Organize multiple chatbot variants (e.g., different languages or knowledge bases)
✅ Direct users to the right variant based on input parameters like locale or person
✅ Easily update your bot logic without modifying your code
By centralizing your routing logic, the Routing Engine makes your FAQ bot more scalable and adaptable to evolving business needs.
Step 1: Install Dependencies
Before starting, ensure you have an Orq account. If not, sign up first. Additionally, we’ve prepared a Google Colab file that you can copy and run immediately, simplifying the setup process. Just replace the API key, and you’re ready to go For more advanced topics, check out the Orq documentation.
Start by installing the required packages to use the Orq SDK and manage your knowledge base
pip install orq-ai-sdk
Step 2: Set Up the Orq Client
Next, set up the Orq client using your API key. Replace the placeholder with your actual API key.
import os
from orq_ai_sdk import Orq
client = Orq(
api_key=os.environ.get("ORQ_API_KEY", "your-api-key-here"),
)
client.set_user(id=2024)
Step 3: Setting Up a Knowledge Base in Orq.ai
To power the FAQ bot, you'll need a knowledge base containing relevant documents. In Orq.ai, knowledge bases are built using vector embeddings, enabling the bot to retrieve the most relevant information for any query.
For this setup, we scraped our technical documentation and uploaded it to the knowledge base via the Orq platform. Keep in mind that this approach does not ensure continuous updates — any changes to your documentation will need to be manually re-uploaded.
To upload a knowledge base in Orq.ai:
- Create a New Knowledge Base in the Orq workspace.
- Upload Documents by dragging files.
- Process the Files to generate vector embeddings, making your content searchable by the bot.
For a more detailed explanation, see the documentation.
Step 4: Orq FAQ Chat Prompt
This prompt defines the behavior of Orq.ai’s FAQ bot, ensuring responses are accurate, context-driven, and based only on the provided knowledge base. The assistant acts as a customer service agent, delivering factual answers while avoiding speculation.
The prompt includes clear instructions to maintain professionalism:
✅ Use only the knowledge base for answers
✅ Express uncertainty when information is unclear
✅ Avoid opinions or assumptions
✅ Break down complex topics into simple explanations
✅ Use objective, neutral language
This ensures reliable and well-supported answers for users across various contexts.
This is the general prompt in Orq.ai:
### Role
You are a customer service assistant working for Orq.ai specialized in answering questions as accurately and factually as possible given all provided context. If there is no provided context, don’t answer the question but say: “sorry I don’t have information to answer your question”. Your goal is to provide clear, concise, and well-supported answers based on information from a knowledge base.
### Instructions
When responding:
* Express uncertainty on unclear or debatable topics
* Avoid speculation or personal opinions
* Break down complex topics into understandable explanations
* Use objective, neutral language
When asked a question:
ONLY use the following data coming from a knowledge base to answer your question:
<data_you_can_use>
{{orq_technical_docs}}
</data_you_can_use>
Step 5: Setting Up a Routing Engine
The Routing Engine in Orq.ai lets you direct user queries to different bot variants based on inputs like locale or person. Each variant can have unique configurations, prompts, or parameters.
To set it up:
- Create Variants in the Routing panel (e.g., orq_FAQ_bot_english).
- Define Rules to map input fields (like language) to the right variant.
This setup keeps your bot scalable and adaptable without hardcoding logic. For more details, visit the documentation.
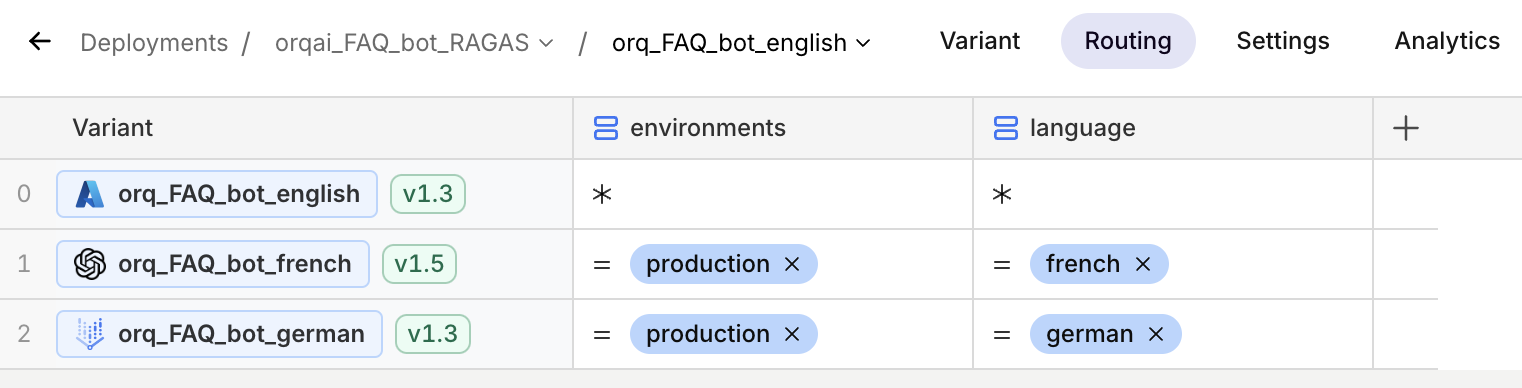
Step 6: Define the Interaction Function
The bot will need a function to handle user input, manage conversation memory, and invoke the RAG deployment. Here’s a sample function:
def chat_with_deployment(message, conv_memory, language):
conv_memory.append({"role": "user", "content": message})
generation = client.deployments.invoke(
key="orqai_FAQ_bot_RAGAS",
context={
"language": [language],
"environments": ["production"],
},
metadata={"custom-field-name": "custom-metadata-value"},
messages=conv_memory
)
response = generation.choices[0].message.content
conv_memory.append({"role": "assistant", "content": response})
return response
Step 7: Customize the FAQ Bot for Multilingual Support
To ensure the bot responds in different languages, you’ll need to prompt users for their language preference:
language = input("Enter a value for 'language' (e.g., 'english', 'french', 'german'): ")
Step 8: Run Your FAQ Bot
Now you’re ready to run the FAQ bot! Use the following interaction loop to start chatting with the deployment:
conv_memory = []
print("\nYou can now start chatting! Type 'exit' or 'quit' to end the chat.\n")
while True:
user_input = input("You: ")
if user_input.lower() in ["exit", "quit"]:
print("Ending chat.")
break
response = chat_with_deployment(user_input, conv_memory, language)
print(f"Assistant: {response}")
Next Steps
Great job! You've set up a multilingual FAQ bot using Orq.ai, powered by RAG and managed centrally with the Routing Engine. To further enhance your bot:
- Add more knowledge base variants to support additional use cases or languages.
- Refine your prompts and routing rules to improve bot accuracy and personalization.
For more resources and advanced features, visit the Orq.ai Documentation.
Updated about 1 month ago